Why should I Dockerize my Node.js app?
You might've heard about the whole Docker thing, but that still doesn't answer the question of why you should bother at all. Well, here's why:
- A Docker image is a self-contained unit that bundles the app with the environment required to run it. No more installing libraries, dependencies, downloading packages, messing with config files, etc. If your machine supports Docker, you can run a Dockerized app, period.
- The working environment of the application remains consistent across workflows and machines. This means the app runs exactly the same for developer, tester, and client, be it on development, staging, or production server.
In short, Docker is the modern-day counter-measure for the age-old "strange, it works for me!" response in software development.
I’ve found this video so useful to get started :
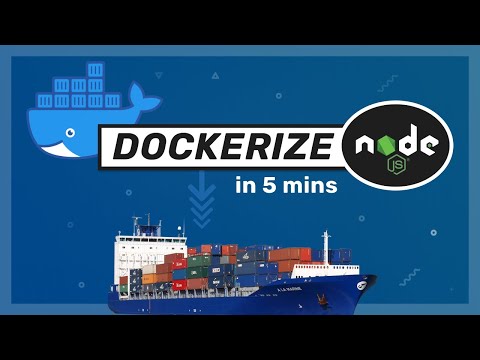
Part 1: Create Node.js app
We'll start by creating a simple Hello World app, a staple of every web developer's portfolio. The app will use Express, one of the most popular Node.js frameworks.
Install Node.js and npm
If you've never worked with Node.js before, start by installing npm – the Node.js package manager. Click here to choose the right version for your environment.
Initialize Project and Install Express.js
Create a new directory for the app and initialize a Node project:
mkdir helloworld cd helloworld npm init
When asked for the details of the application, set the name to
helloworld
. For other options, just confirm the default values with enter.Npm will create a
package.json
file that will hold the dependencies of the app. Let's add the Express framework as the first dependency:npm install express --save
The file should look like this now:
{ "name": "helloworld", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "author": "", "license": "ISC", "dependencies": { "express": "^4.17.1" } }
TableMain of Hello World
With everything installed, we can create an
index.js
file with a simple HTTP server that will serve the Hello World app://Load express module with `require` directive var express = require('express') var app = express() //Define request response in root URL (/) app.get('/', function (req, res) { res.send('Hello World!') }) //Launch listening server on port 8081 app.listen(8081, function () { console.log('app listening on port 8081!') })
Run the app
The application is ready to launch:
node index.js
Go to
http://localhost:8081/
in your browser to view it.Part 2: Dockerize Node.js appplication
Every application requires a specific working environment: applications, dependencies, databases, libraries, everything in a specific version. Docker allows you to create such environments and pack them into a container.
Contrary to a VM, the container doesn't hold the whole operating system — just the applications, dependencies, and configuration. This makes Docker containers much lighter and faster than regular VMs.
In this part of the guide, we will build a Docker container with the Hello World app, and then launch the app in a Docker container.
If you didn't configure the app in the first part of the guide, you can fork a working Node project from our repository on GitHub.